FastAPI Helpers
FastAPI Helpers module contains wrapper classes for FastAPI routes, routers and apps.
| from backend_dev_utils import FastAPIApp
app = FastAPIApp(title="My FastAPI App", version="1.0.0")
|
Assuming that you put this code snippet in main.py, you can run it by:
fastapi run main.py
And this is what you will get at "http://localhost:8000/docs":
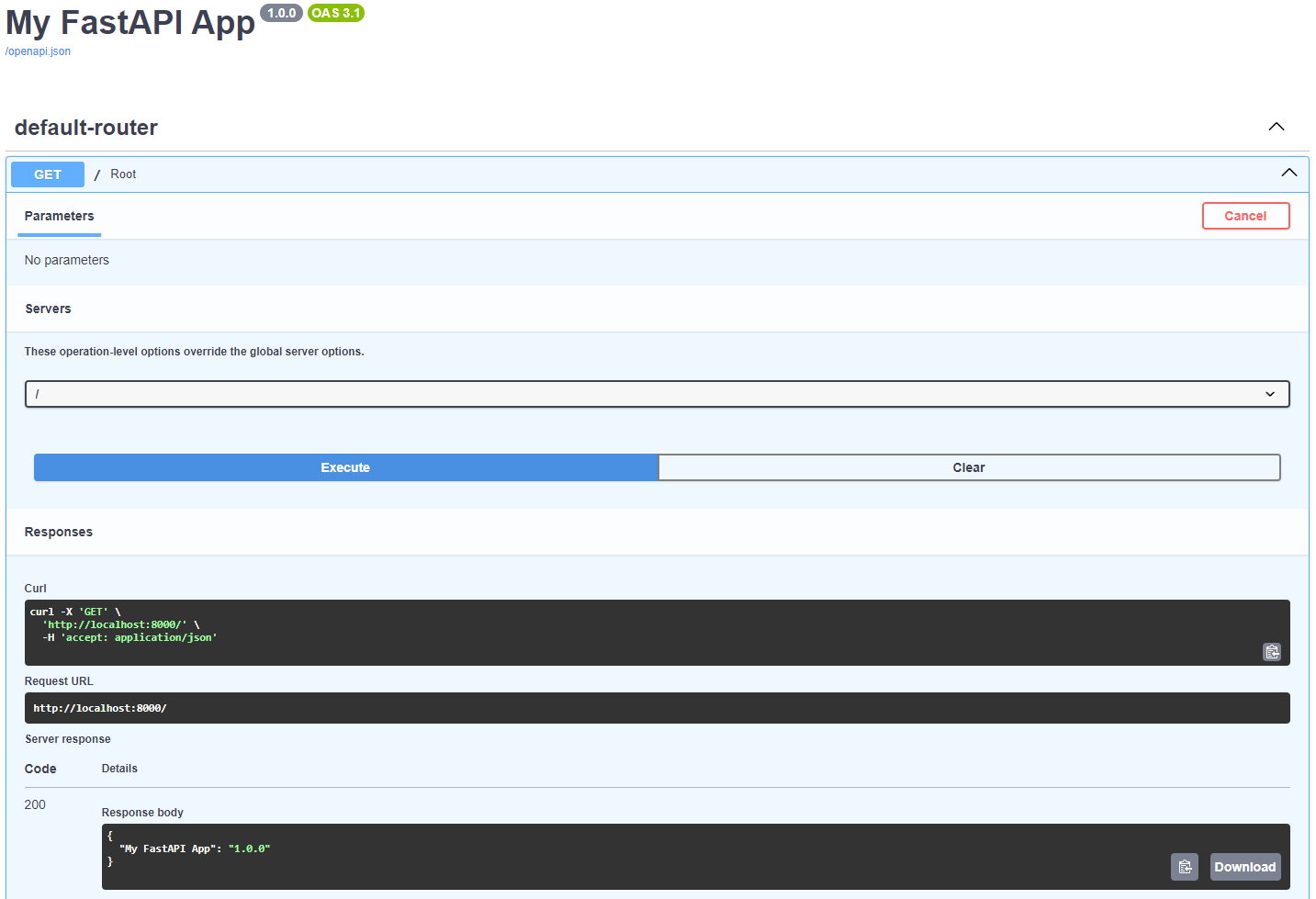
This was a basic application that contains only the default route that I include in the app. But if you don't want to include it, and create your own routers and routes:
| from backend_dev_utils import FastAPIApp, FastAPIRouter, FastAPIRoute
def hello_endpoint(name: str):
return {"message": f"Hello {name}"}
hello_route = FastAPIRoute(path="/hello", endpoint=hello_endpoint, methods=["GET"])
my_router = FastAPIRouter(prefix="/api/v1", tags=["my-router"])
my_router.add_route(hello_route)
fastapi_app = FastAPIApp(
routers=[my_router],
title="Hello API",
description="An API to say hello.",
version="0.0.1",
include_default_router=False,
)
app = fastapi_app.app
|
And when you run it, you will get:
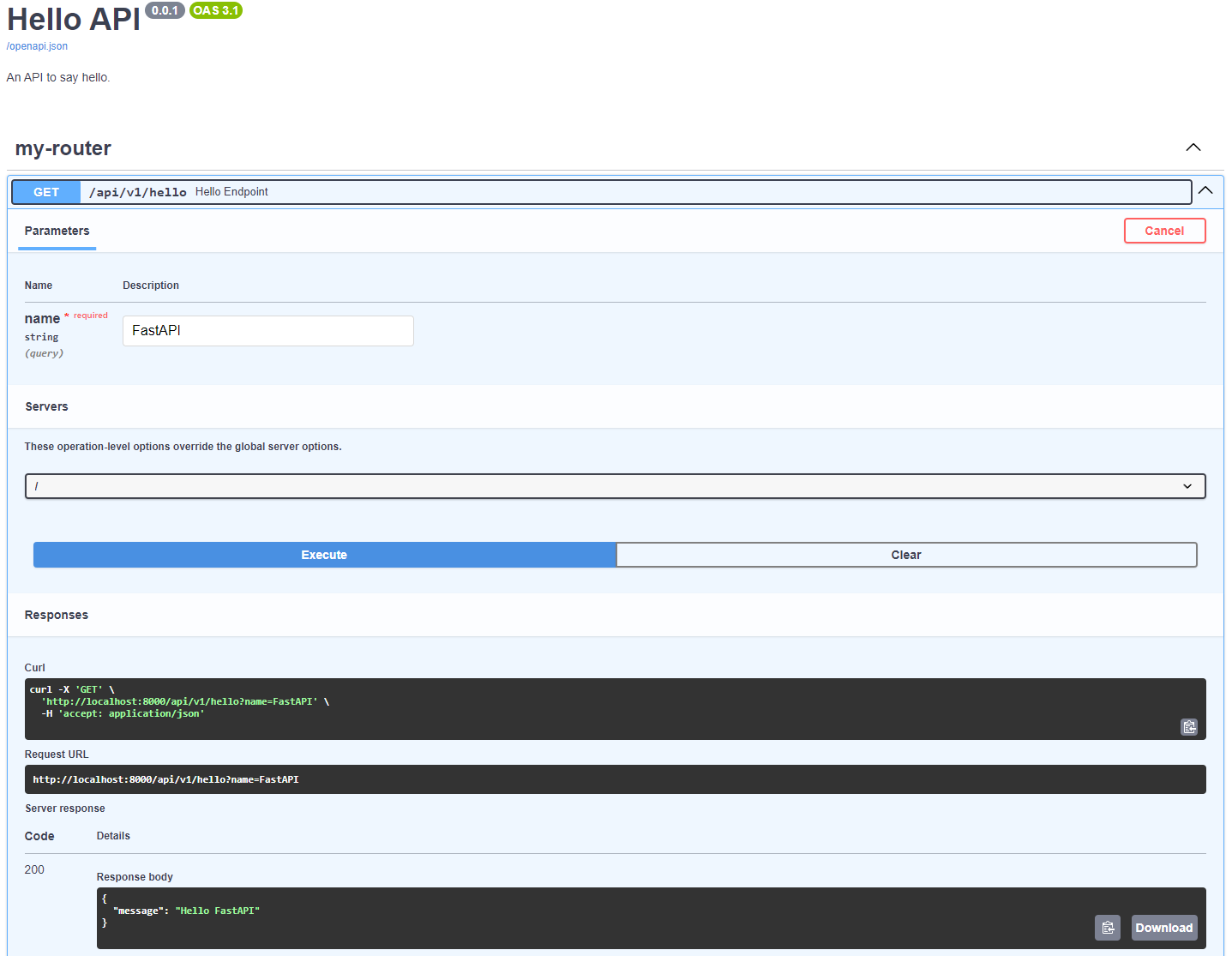
Or you can add the FastAPIRoute object directly to the FastAPI app as:
| from backend_dev_utils import FastAPIApp, FastAPIRouter, FastAPIRoute
def hello_endpoint(name: str):
return {"message": f"Hello {name}"}
hello_route = FastAPIRoute(path="/hello", endpoint=hello_endpoint, methods=["GET"])
fastapi_app = FastAPIApp(
routes=[hello_route],
title="Hello API",
description="An API to say hello.",
version="0.0.1",
include_default_router=False,
)
app = fastapi_app.app
|